[HELP] Java Code
By icebuken
@icebuken (28)
Philippines
August 11, 2010 8:49am CST
hi to all my fellow esf coders and programmers,
i am here to ask for your wonderful ideas and advices in regards to my java code. this java code deals with separate java classes having different implementations and this java code also deals with text-based database manipulation. (yeah yeah i know that text-based database are not good but it's for school demonstration purposes only so please bear with me :D..
enough with the chitchat and let's start with the meat of the discussion. here is my login class:
login class code:
//login class
import java.io.*;
import javax.swing.*;
public class LoginFileRead {
public boolean searchUsername(String user, String pass){
String myf="login.txt";
String content=null;
String username="";
String password="";
Boolean bool=false;
File f=new File(myf);
if(f.exists()!=true){
JOptionPane.showMessageDialog(null,"File Not Found");
System.exit(0);
}
try{
BufferedReader br=new BufferedReader(new FileReader(myf));
while((content=br.readLine())!=null){
username=content.substring(0,content.indexOf(",")).trim();
password=content.substring(content.indexOf(",")+1,content.length()).trim();
if((user.equals(username)) && (pass.equals(password))){
bool=true;
break;
}else{
bool=false;
}
}
if(bool==true){
JOptionPane.showMessageDialog(null,"Welcome");
}else{
JOptionPane.showMessageDialog(null,"Invalid Username or Password");
}
}
catch(Exception e){
JOptionPane.showMessageDialog (null, "Invalid Input");
}
return bool;
}
}
and here is my java login.txt file reader code
login file reader
//login file reader class
import java.io.*;
import javax.swing.*;
public class LoginFileRead {
public boolean searchUsername(String user, String pass){
String myf="login.txt";
String content=null;
String username="";
String password="";
Boolean bool=false;
File f=new File(myf);
if(f.exists()!=true){
JOptionPane.showMessageDialog(null,"File Not Found");
System.exit(0);
}
try{
BufferedReader br=new BufferedReader(new FileReader(myf));
while((content=br.readLine())!=null){
username=content.substring(0,content.indexOf(",")).trim();
password=content.substring(content.indexOf(",")+1,content.length()).trim();
if((user.equals(username))&& (pass.equals(password))){
bool=true;
break;
}else{
bool=false;
}
}
if(bool==true){
JOptionPane.showMessageDialog(null,"Welcome");
}else{
JOptionPane.showMessageDialog(null,"Invalid Username or Password");
}
}
catch(Exception e){
JOptionPane.showMessageDialog(null, "Invalid Input");
}
return bool;
}
}
and these is my java main method code
main method:
//main method class
public class StartUp{
public static void main(String args[]){
new Login();
}
}
my java code is already working but it seems to me that my code is not that optimized so what i want to happen here is to optimize my java code for better and faster performance. so what do i need to do in order to optimize my java code? i mean is there any way to shorten my code? your help will be much appreciated. thank you in advance to those who will respond to my query and please don't flame me :D m just a newbie when it comes to coding :D
here is my login.txt file or you can just create your own login atabase file. i used a comma (",") as a delimiter. this is the content of my login.txt database file:
already, there
iamthere, notyet
hi, hello
where the first word is the username and the second word just right after the comma (",") --my delimiter-- is the password..i hope you guys got what i meant :D thanks again :D
1 response
@Q_Savvy (131)
• India
4 Nov 10
I would paste some code, but I can't since my Rating isn't 500.. blegh!
Anyway,
where you've given the command to set "bool = true" , you could simply display the JOPtionPane directly there, followed by "return true;". Follow the same approach for the "bool = false", followed by "return false;". That way you won't need to use the "bool" variable.
If you make the above change, you could also remove the condition "(if bool==true).." etc.
Another point to be noted is that instead of importing java.io.*; only import packages that are required, because using .* imports every package available for io, 99% of which you don't need.
If you're using Eclipse IDE, you can remove the 2 import statements, and let the errors pop up. Then hover your mouse over the error and it will recommend a package to be imported. Import it.
Hope that helps! :)
@Q_Savvy (131)
• India
4 Nov 10
Oh I forgot to add - you won't notice any difference with such minute optimization unless the code extends to about 5000 lines at least, OR you have about a 2GB data containing database. Although it's a small optimization technique and doesn't seem to do much here, in the cases that I mentioned above it works wonders!
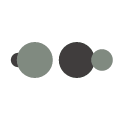